How to Create a Mutation Query of Create a user in Graphql || Nestjs + Typeorm + Mongodb
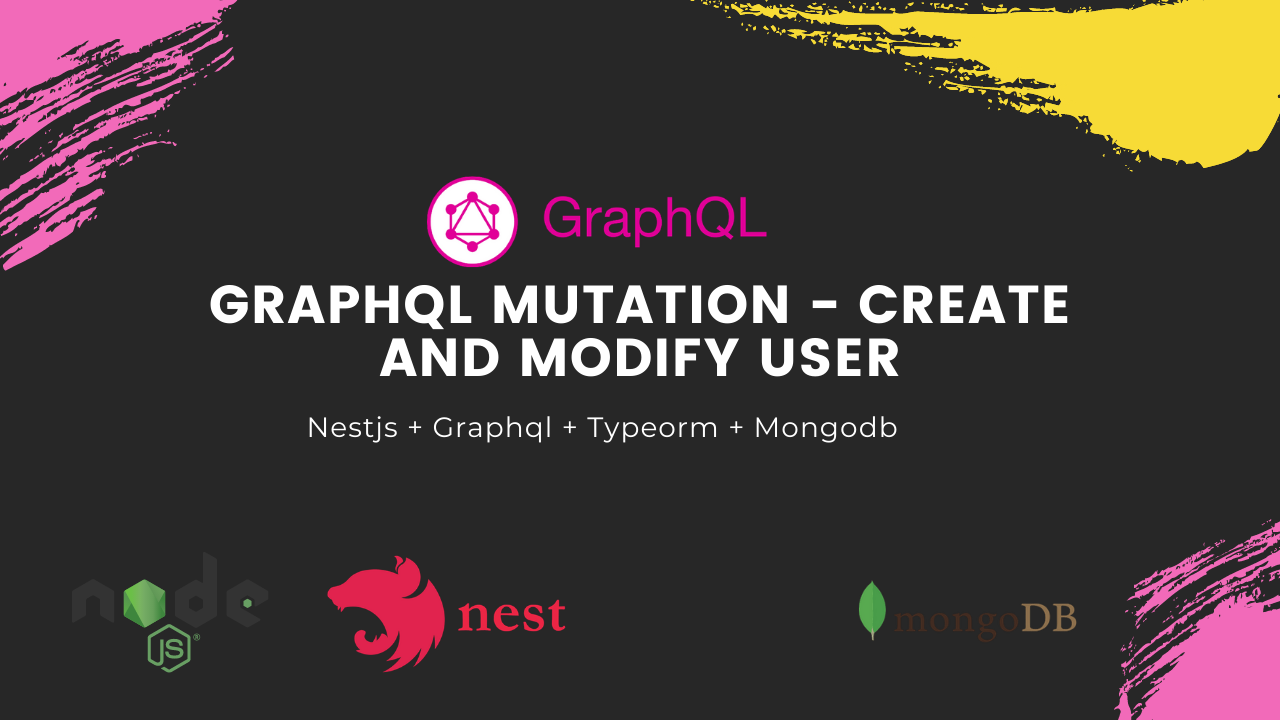
In this blog, we will discuss how to make a Mutation on Graphql
to Create and Modify a user. We only gonna modify 2 files, user.service and user.resolvers
- user.service
- user.resolver
Mutation: Create User
user.service
We will create one function here to create a user, we’ll name it ‘createUser(userData)
‘. We have one parameter here containing all of our input data that required to create a user. the example of our input data is slug, name, and email. The main code here is to create a document inside of our user. That will be done by one block of code of Typeorm<yourUserRepository>.insert(<yourUserObject>)
Example: this.userRepository.insert(userData)
Although before that, we have to check if the inputted data is already an existing user or not. In pseudocode, here our simple approach :
Find in userRepository.find(slug: userData.slug)
If (found)
Throw error
Else
userRepository.insert(userData)
Our finish example of createUser function in user.service look like this:
async createUser(userData) {
const existingUser = await this.findBySlug(userData.slug);
if (existingUser) {
const error = new Error('User exist already!');
throw error;
}
await this.userRepository.insert(userData);
return this.findBySlug(userData.slug)
}
user.resolver
Next, we’re gonna modify our code in user.resolver. we’re gonna create a Mutation Query that makes a user and return it. For the demonstration, we’re gonna take 3 arguments, which are email, name, and slug(unique username). We’ll bundle this data into userData and pass it into our createUser function in user.service. This userData was the inputted data so we can make ourselves @InputType() as a declaration. Our resolvers pretty much look like the code below:
@InputType()
export class userInput {
@Field({ nullable: false, description: 'Unique username' })
slug?: string;
@Field({ nullable: true, description: 'Full name' })
name?: string;
@Field({nullable: true, description: 'User Email' })
email?: string
}
@Mutation(returns => User)
async editUser(
@Args('editUserData') editUserData: userInput) {
return await this.usersService.editUser(editUserData);
}
Mutation: Modify User
user.service
async editUser(editUserData) {
const existingUser = await this.findBySlug(editUserData.slug);
if (!existingUser) {
const error = new Error('User doesnt exist');
throw error;
}
const temp = await this.userRepository.update({slug: editUserData.slug}, editUserData);
return this.findBySlug(editUserData.slug);
}
user.resolver
@Mutation(returns => User)
async editUser(
@Args('editUserData') editUserData: userInput) {
return await this.usersService.editUser(editUserData);
}